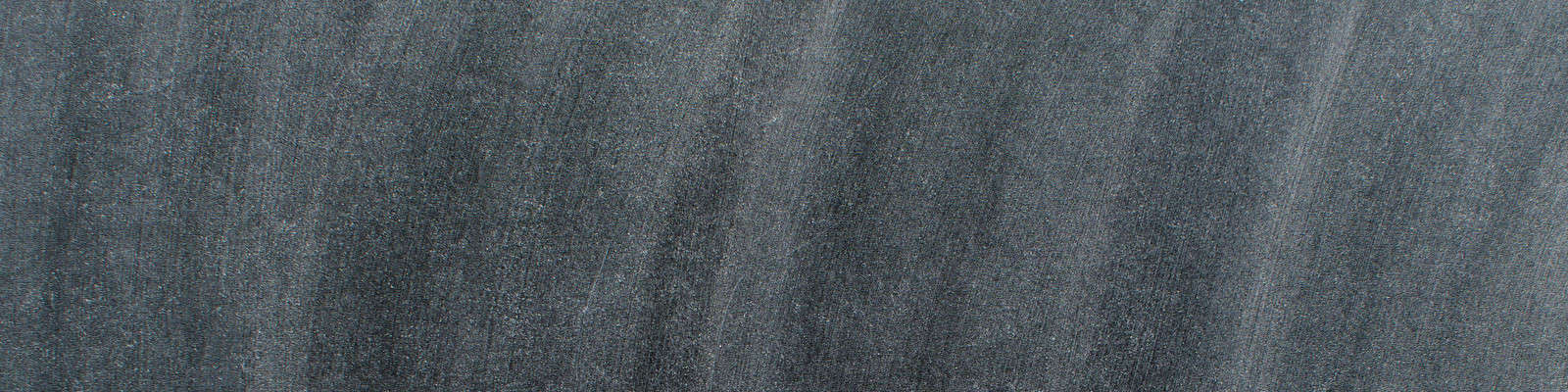
SQL Where Clause
PLEASE NOTE: This tutorial comes from my FREE video course called SQL Boot Camp.
-- drop table posts;
create table posts (
id integer,
title character varying(100),
content text,
published_at timestamp without time zone
);
insert into posts (id, title, content, published_at) values (100, 'Intro to SQL', 'Epic SQL Content', '2018-01-01');
insert into posts (id, title, content, published_at) values (101, 'Intro to PostgreSQL', 'PostgreSQL is awesome!', now());
insert into posts (id, title, content) values (102, 'Intro to SQL Where Clause', 'Easy as pie!');
-- equality
select * from posts where title = 'Intro to SQL';
select * from posts where id = 101;
-- inequality
select * from posts where id != 101;
select * from posts where id <> 101;
select * from posts where not id = 101;
-- multiple values
select * from posts where id in (100,101);
-- >, >=, <, <=
select * from posts where id > 101;
select * from posts where id >= 101 ;
-- nulls
select * from posts where published_at >= '2018-01-15';
select * from posts where published_at < '2018-01-15';
select * from posts where published_at is null;
select * from posts where published_at is not null;
-- pattern
select * from posts where content like '%pie%';
select * from posts where content like '%PIE%';
select * from posts where content ilike '%PIE%';
-- and / or / between
select * from posts where id = 100 and title like '%SQL';
select * from posts where id = 100 or published_at is null;
select * from posts where published_at >= '2018-01-15' and published_at < '2018-02-15';
select * from posts where id between 100 and 101;
Please go ahead and leave a comment below if you have any questions about this tutorial.
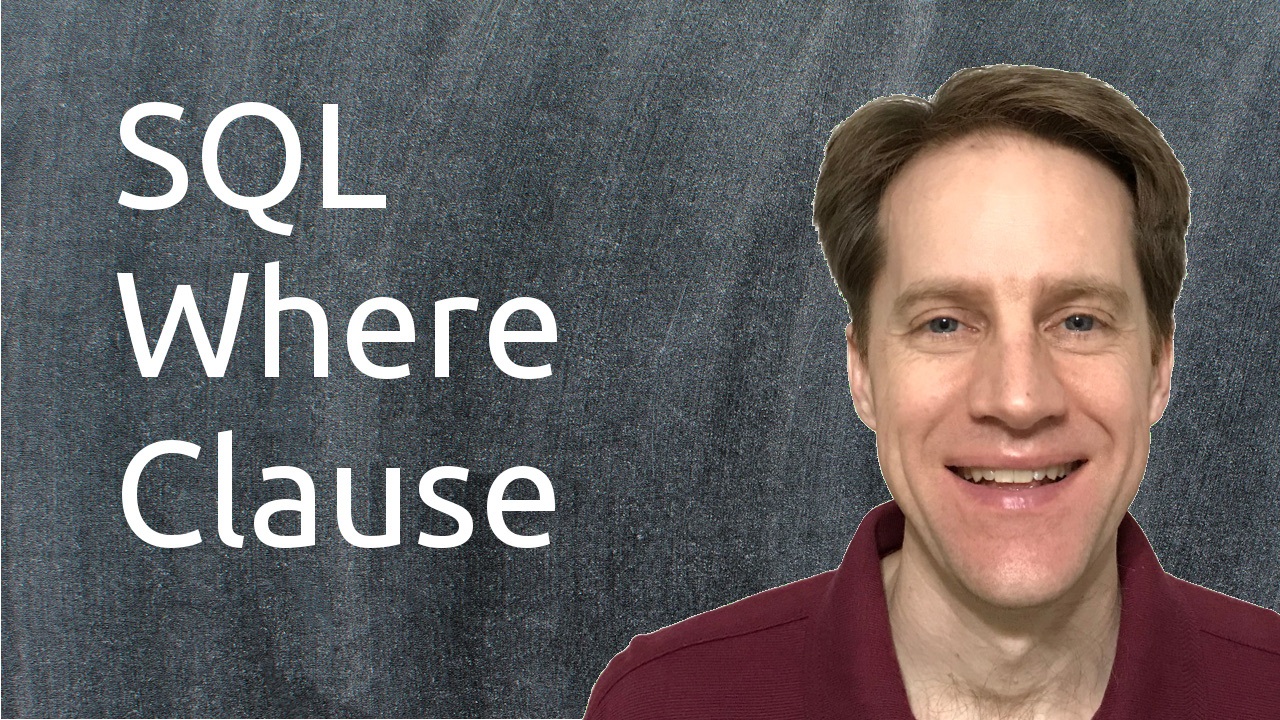